So I got one of these Cheap Chinese HID RGB LEDs the other day. The include software only interfaced with Outlook, sigh. Oh well, lets start poking around.
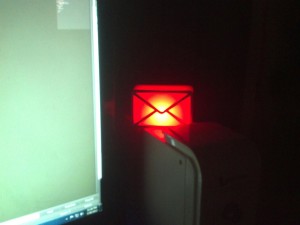
I found that the drive is for an HID (Human Interface Device) device so I opened python and grabbed pywinusb, which includes a HID helper class.
So again looking at the driver, I found that the vendor-id was 0x1294. This number 4278190081 for the report… I don’t remember how I found it…
But after finding out those two values I started throwing bits at it. I sent 0x0001 and to my surprise, the envlope started glowing green. There are 8 settings, and the mapping is as follows:
- 0 – off
- 1 – green
- 2 – red
- 3 – blu
- 4 – teal
- 5 – yelloish-green
- 6 – purple
- 7 – white(ish)
Bam… Disco Party In My Room
import time,random import pywinusb.hid as hid def get_device(): all_devices = hid.HidDeviceFilter(vendor_id = 0x1294).get_devices() if len(all_devices)==0: print "Can't find target device" else: return all_devices[0] return null def set_color(device,color): for report in device.find_output_reports(): if 4278190081 in report: report[4278190081] = [color,0,0,0,0] report.send() if __name__ == '__main__': try: d = get_device() d.open() set_color(d,0) color = 0 while True: nucolor = color # windows has a bad random, while color is nucolor: # i saw a ten streak of 6's nucolor = random.randint(1, 7) # so this forces it to change color = nucolor print color set_color(d,color) time.sleep(1) finally: d.close() exit()
Ok, Lets now grab my Reddit Inbox.
I quickly found the reddit_api python module, now called PRAW (Python Reddit API Wrapper), but it didn’t quite do what I wanted. [*Disclaimer: This might not be true with newer versions of PRAW]
So I forked it.
My changes include a direct way to access reddits unread message end-point.
With that in hand, we can build a script to check our unread messages.
#configure your details here user = "" #reddit username password = "" #reddit password delay = 60*5 #delay in seconds between checking mail #dont you dare look down past here!!! import time import pywinusb.hid as hid import reddit def get_device(): all_devices = hid.HidDeviceFilter(vendor_id = 0x1294).get_devices() if len(all_devices)==0: print "Can't find target device" else: return all_devices[0] return null def set_color(device,color): for report in device.find_output_reports(): if 4278190081 in report: report[4278190081] = [color,0,0,0,0] report.send() if __name__ == '__main__': try: d = get_device() d.open() set_color(d,0) r = reddit.Reddit(user_agent="redmail") r.login(user=user,password=password) inbox = r.get_inbox() while True: new = inbox.get_new_messages(force=True) if len(new) > 0: set_color(d,2) else: set_color(d,0) time.sleep(delay) finally: d.close() exit()
And done, Reddit orangered’s on my desk.
The Github project: https://github.com/willwharton/redmail